In my last blog post I introduced you to Zumero, an Enterprise solution, that allow you to replicate and sync SQL Server data across all your team's mobile devices.
I had the pleasure of meeting up with Zumero founder, Eric Sink, @eric_sink at the recent SQL Saturday event in Copenhagen.
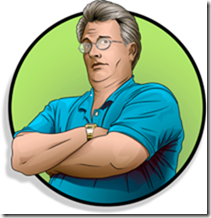
We agreed to do a short interview by mail, so I could share my Zumero questions with my blog readers. So without further ado, let’s get started.
I know you have been involved with other software products (SourceGear). What is the story behind Zumero?
We think of Zumero as a new start-up that is incubating inside SourceGear, so there is much shared history.
We started SourceGear in 1997, initially as a contracting shop building custom software for mobile devices. We did a lot of work with Blackberry devices and some very early Motorola smart phones.
Then we got into version control tools, which has been a great business for us over the last 15 years. We are still serving a large customer base with on-going active development of SourceGear Vault.
But in the last few years, the world of mobile has been drawing us back in. :-)
And from a certain point of view, our work on version control means that we have been doing "sync" for 15 years.
And SourceGear Vault has always been built on SQL Server.
So that's how we got the three main ingredients of Zumero: SQL Server, mobile, and sync.
Some of the features that Merge Replication provide are: - Minimal client code, secure, compressed transfer over https, server side filtering, read-only tables, conflict handling. How does Zumero stack up?
I /think/ we stack up pretty well, although we still have many ideas for how to make our product better.
"minimal client code" -- The Zumero Client SDK tries to make everything easy. Just call the Zumero sync function and Zumero will synchronize changes in both directions.
"secure" -- Zumero is designed to work over SSL/https. The protocol includes authentication. And on the client, Zumero is compatible with bulk encryption libraries such as SQLCipher.
"compressed transfer over https" -- All packages exchanged between Zumero client and server are compressed. In fact, the packages from server to client are often "deltified" to make them very small indeed.
"server side filtering" -- Zumero does support the ability to exclude tables, or columns from tables, or to filter rows on a custom WHERE clause.
"read only tables" -- This can be accomplished with Zumero's authentication and permissions.
"conflict handling" -- Zumero handle conflict resolution on the server. The default rules seem to be appropriate for most cases, but those rules can be customized to do whatever is needed for a given situation.
In addition, Zumero adds a few things that SQL CE merge replication does not, most notably, support for iOS and Android devices.
OTOH, Zumero is built on SQLite, which does not resemble SQL Server as closely as SQL CE does. We are constantly working to provide Zumero customers with a smooth experience by improving features, tooling and documentation. My blog series on the differences between SQL Server and SQLite is a part of those efforts:
Rob Tiffany has demonstrated scaling Merge Replication. How does Zumero scale?
This is one area where Zumero's youth is evident, and I shall not pretend. There is much more experiential knowledge about scaling with merge replication. Rob Tiffany's "cheat sheet" is a gold mine of information:
And some of his advice would be applicable to Zumero as well.
However, we are quite happy with the scalability results we have achieved so far and we continue to push further.
(a) We do a lot of "crowd testing", using lots of clients to abuse the Zumero server so we can tune it for reliability and performance.
(b) We are currently working with some customers who are integrating Zumero into an environment where merge replication is already in use (as a scaling solution for the SQL Server backend, not as a mobile sync solution). We have some additional testing to do here, but we hope to able to publish some guidelines about the compatibility of Zumero with this kind of situation.
(c) Finally, we have tested and verified another way of scaling out, by having multiple Zumero servers talking to a single instance of SQL Server. This can make a significant difference and is not difficult to set up behind a round robin DNS.
You are using Triggers and tracking tables on SQL Server, and not the built-in Change Tracking. Why?
The real reason is that Zumero's core sync code existed before we made the decision to make SQL Server the primary focus of the product.
That said, the documentation for Change Tracking raises questions for us. We wonder if it would require changes to our sync algorithm. We wonder if our support for tracking schema changes would still work. We wonder about the apparent dependence on snapshot isolation.
We may explore this further in the future, but right now, we've got a solution which is robust, so we don't feel much pressure to change it.
Do you provide a Windows Mobile (.NET Compact Framework) client library?
Currently, no, but we are planning to do so. The implementation work for Windows Mobile 6 support is done, but it has not yet been released as part of the product. If a Zumero customer needs this, we would be ready to discuss and coordinate making it available.
What do you recommend for use as data access API towards SQLite on Windows platforms?
Right now, there is no API we can recommend without caveats. Or rather, there are several good choices, but no clear winner.
For desktop Windows only, the core SQLite team products System.Data.SQLite, an ADO.NET provider which is fully compatible with EF6. If you're not using mobile, this is almost certainly the best choice.
Frank Krueger's sqlite-net wrapper is quite popular, and for some very good reasons. But last I knew, none of the various PCL forks have been folded back in, and that has been an issue for some. Nonetheless, this is the wrapper we encounter most often.
WinRT without System.Data is a story with a big hole in it. We remain hopeful that Microsoft has some more good stuff in the pipeline.
Looking beyond Windows to include iOS and Android as well, things can get complicated. Both of these other platforms include a [different] version of SQLite as part of the mobile OS. Many apps use those. Some bundle their own. Some replace SQLite with SQLCipher. Things can get tricky. In addressing this set of problems with our customers, we've built some stuff that we are planning to make available to the community as open source (to be announced on my blog, soon, I hope). [Ed: Available as source code on Github, and NuGet packages coming soon]